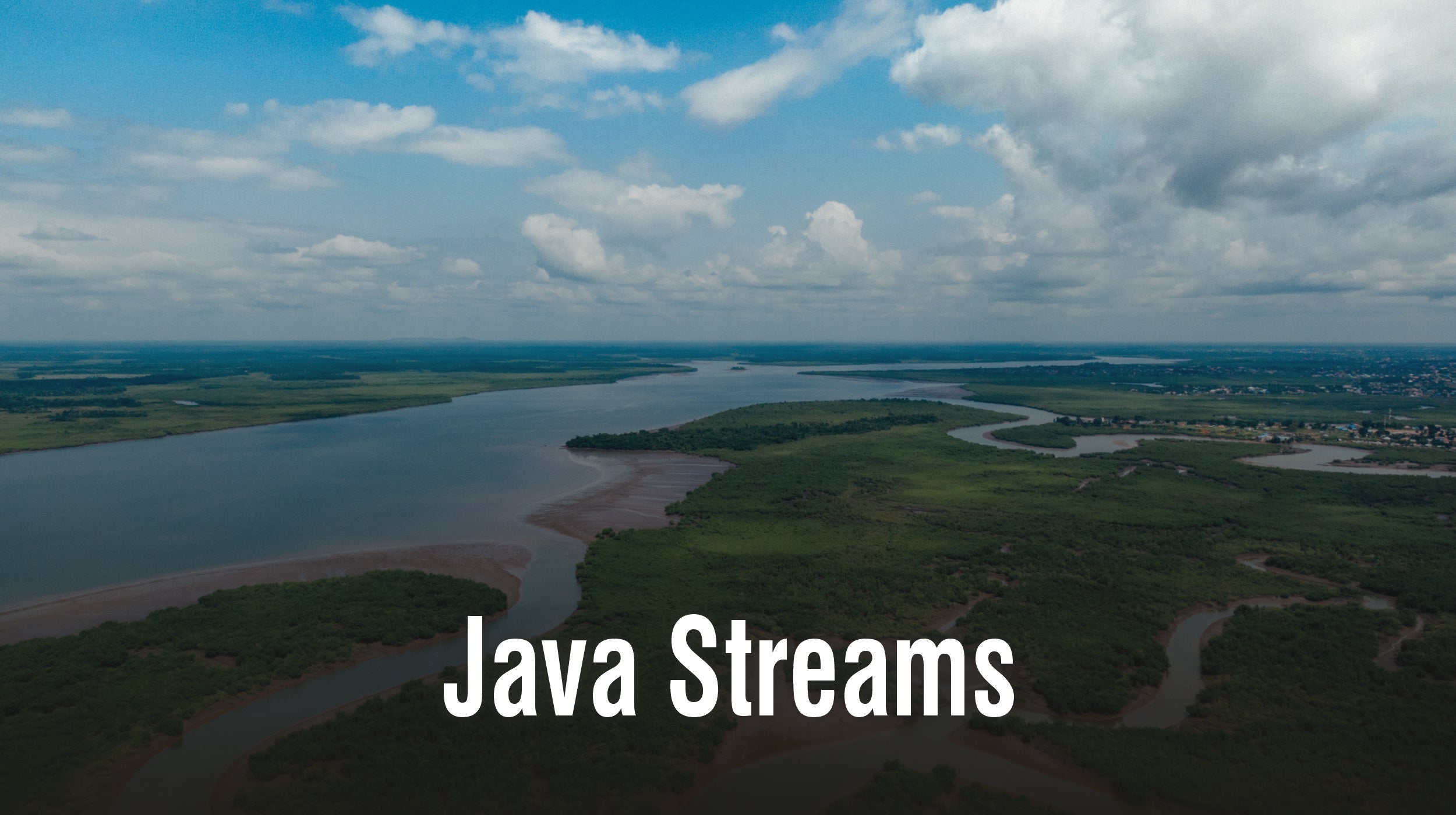
Java Streams
Introduction
This blog talks about Java streams. The stream is a new addition in Java 8 that gives much power to Java while processing data. One thing to note here is that the stream mentioned here has nothing to do with Java I/O streams. This Java tutorial will focus on the Java 8 Streams in detail.Streams make processing a sequence of elements of the same datatype a cakewalk. It can take input in the form of any collections in Java, Arrays, Java I/O streams, etc. The interesting fact here is that you don’t need to iterate through these inputs to process each element. Automatic iterations are a built-in feature of the stream.
In this blog, we will learn to create a stream and look at how stream operations in Java function. We will also take a look at improvements made by Java 9 on the stream interface.
Creating a Stream:
Let’s see how a stream can be declared in Java 8.
First, you will import java.util.stream class to use the Java 8 Stream feature. Now assuming we have an array ‘arrayOfCars’ as the input source to the stream,
private static Car[] arrayOfCars = { new Car(1, “Mercedes Benz”, 6000000.0), new Car(2, “Porsche”, 6500000.0), new Car(3, “Jaguar”, 7000000.0) }; |
The stream can be created as given below:
Stream.of(arrayOfCars); |
If we are having a List, a stream can be created by calling the stream() method of that list itself.
sampleList.stream(); |
We can also create Stream from different objects of a collection by calling the Stream.of() method.
Stream.of (arrayOfCars[0], arrayOfCars[1], arrayOfCars[2]); |
Or just by using the Stream.builder() method.
Stream.Builder carStreamBuilder = Stream.builder(); carStreamBuilder.accept( arrayOfCars[0] ); carStreamBuilder.accept( arrayOfCars[1] ); carStreamBuilder.accept( arrayOfCars[2] ); Stream carStream = carStreamBuilder.build(); |
Stream Operations:
We have seen the different ways of creating a stream. Now let us have a look into various Stream operations.The main use of stream is to perform a series of computations operations on its elements until it reaches the terminal operation.
Let’s look at an example:
List flowers =
Arrays.asList(“Rose”, “Lilly”, “Aster”, “Buttercup”, “Clover”);
flowers.stream().sorted().map(String::toUpperCase).forEach(System.out::println);
|
Here the stream performs sorting and mapping operations before iterating and printing each element in the stream. So we can understand that the stream operations are either intermediate or terminal. In intermediate operations, the output will be stream format itself.

Intermediate stream operations:

Terminal stream operations:

Improvement made by Java 9 on stream interfaces
- Java 9 has added a stream.ofNullable() method to the stream interface. It helps to create a stream with a value that may be null. So, if a non-null value is used in this method, it creates a stream with that method; otherwise, it creates an empty stream.
- Both the methods takewhile() and drpwhile() can be operated in Java stream. They are used to obtain a subset of the input stream.
- Java 9 has added an overloaded version of the Stream.iterate() method that accepts a predicate and terminates the stream when the condition specified is true.
End note:
In this blog, we learned about the improvements made by Java 9 on stream interfaces. We also studied Java 8 stream features in detail—how to create a stream, stream operations, the uses of a stream, among other topics. Next, we learned about different stream intermediate operations and stream terminal operations and briefly looked at how Java stream provides a functional style of programming to Java besides its Object-oriented programming style. It is important to learn and understand this newly-added feature of a stream while studying Java programming.
Get one step closer to your dream job!
Prepare for your Java programming interview with Core Java Questions You’ll Most Likely Be Asked. This book has a whopping 290 technical interview questions on Java Collections: List, queues, stacks, and Declarations and access control in Java, Exceptional Handling, Wrapper class, Java Operators, Java 8 and Java 9 etc. and 77 behavioral interview questions crafted by industry experts and interviewers from various interview panels.
We’ve updated our blogs to reflect the latest changes in Java technologies.
This blog was previously uploaded on April 2nd, 2020, and has been updated on January 7th, 2022.
Share