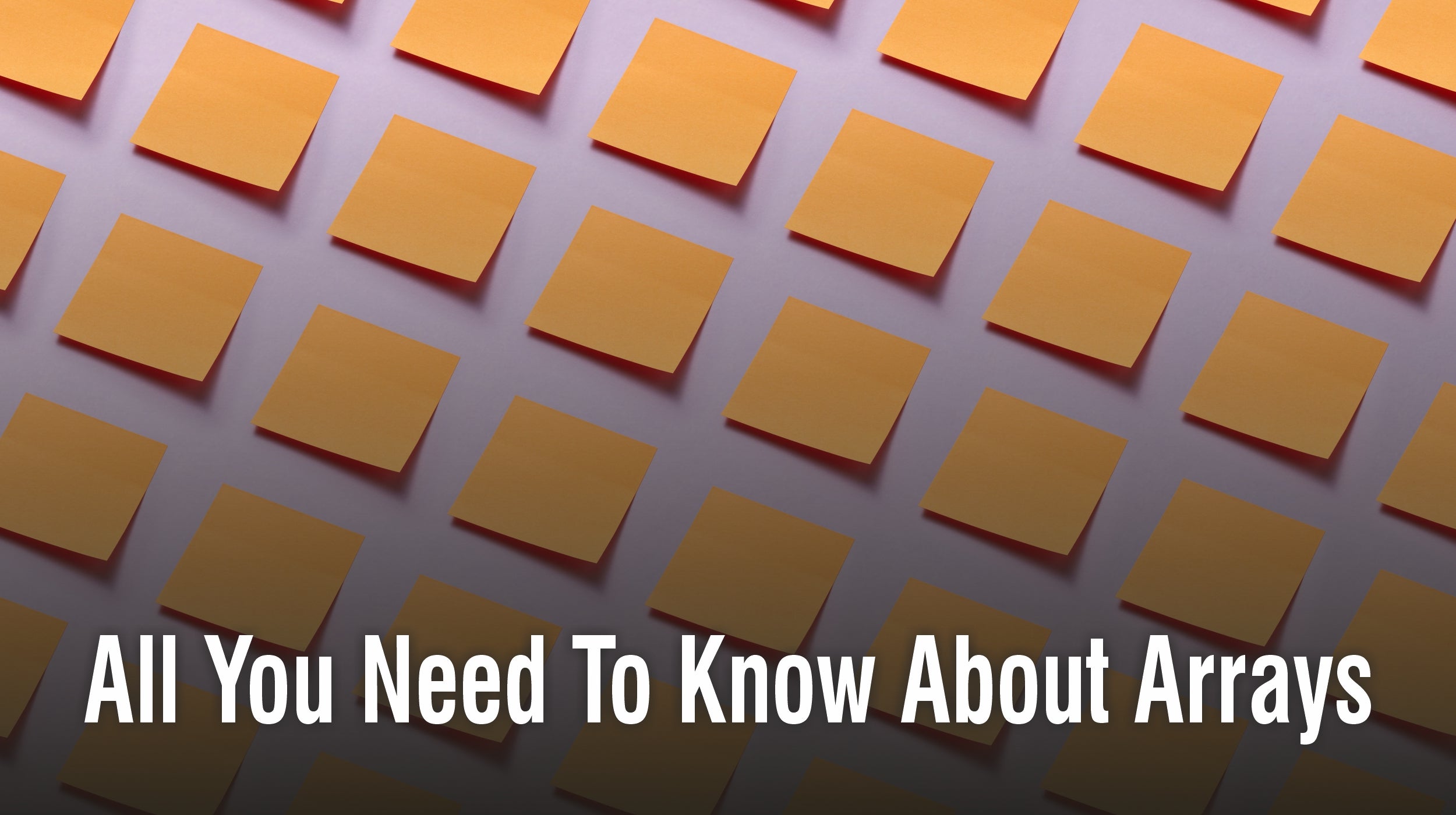
All You Need To Know About Arrays
An array is a fixed collection of items of the same data type represented by a single variable. We can access the sequential Data Structure item called ‘element’ using an array index. Arrays can be single-dimensional or 2 dimensional with rows and columns.To reach node E we start from node A and move downwards, as deep as we can go. We will reach node C. Now, we backtrack to node B, to go downwards towards another adjacent vertex of B. In this way, we reach node E.
For example: int myarray[5];
We can initialize an array either during declaration or later.
For example: type myarray[size] = {values/elements}
Arrays can be declared in many ways depending on the programming language used. For example, a string in C is represented by a character array. Here, we have declared and initialized a character array.
char charArray = {‘ H ’,’ E ’,’ L ’,’ L ’,’ O ’}.
We can declare an array of Strings in the following way:
String names[3] = {“John”,”Kelvin”,”Simmons”}
Array index [0] John
Array index [1] Kelvin
Array index [2] Simmons
Let’s see how each operation works.
int arr[5]={10,9,8,6} insert 7 at arr[3]
Start traversing from the last position and move to the next until you reach the 3rd position. Remember, arr[0] is the 1st element. We need to reach arr[3] which has value 6 in our example. Move the arr[3] value to the right and insert 7 in arr[3].
Below is a pseudo code to indicate the same :
Check out the books we have, which are designed to help you clear your interview with flying colors, no matter which field you are in. These include HR Interview Questions You’ll Most Likely Be Asked (Third Edition) and Innovative Interview Questions You’ll Most Likely Be Asked.


An array is used to implement other Data Structures such as Stacks, Queues and Lists.
How is an array represented?
You can define integer arrays, string arrays or object arrays. Array size has to be defined when it is declared. This ensures memory is allocated based on the array size.For example: int myarray[5];
We can initialize an array either during declaration or later.
For example: type myarray[size] = {values/elements}
Arrays can be declared in many ways depending on the programming language used. For example, a string in C is represented by a character array. Here, we have declared and initialized a character array.
char charArray = {‘ H ’,’ E ’,’ L ’,’ L ’,’ O ’}.
We can declare an array of Strings in the following way:
String names[3] = {“John”,”Kelvin”,”Simmons”}
Array index [0] John
Array index [1] Kelvin
Array index [2] Simmons
Array Operations:
An array supports the following basic operations:- Insertion – insert an array element at the index and ensure there is no overflow
- Deletion – delete an array element at the index
- Traverse – loops through and prints all the array elements sequentially
- Search – search by using an array index or by the value
- Update – update the array element of the index
Let’s see how each operation works.

Array Insertion and Traversal:
You can insert one or more elements into an array, at the beginning, end or any index. Let’s see the steps of inserting an array element at index 3.int arr[5]={10,9,8,6} insert 7 at arr[3]
Start traversing from the last position and move to the next until you reach the 3rd position. Remember, arr[0] is the 1st element. We need to reach arr[3] which has value 6 in our example. Move the arr[3] value to the right and insert 7 in arr[3].
Array deletion and Traversal:
Deletion at the beginning and end of the array is straight forward. However, when you have to delete from the middle of the array, rearrange the element to occupy the space that has been emptied.
Let’s take the same example as above and delete the element with value 8 which is arr[2].
Traverse through arr[3] and delete the value of arr[3]. This value now becomes null. Go to the next index arr[4] and move this element to the 3rd position. Stop the move operation if this is the end of the array.
The new array now has these elements: {10,9,6}
Array Search:
We can search an array for a specific element using the index or value. When you traverse through an array in a loop iterators reference the element and help to move to the next position.Below is a pseudo code to indicate the same :
for(iter=0;iter<n;iter++) { if ( arr[iter]== item) Return iter; } |
Get one step closer to your dream job!
Check out the books we have, which are designed to help you clear your interview with flying colors, no matter which field you are in. These include HR Interview Questions You’ll Most Likely Be Asked (Third Edition) and Innovative Interview Questions You’ll Most Likely Be Asked.
Share