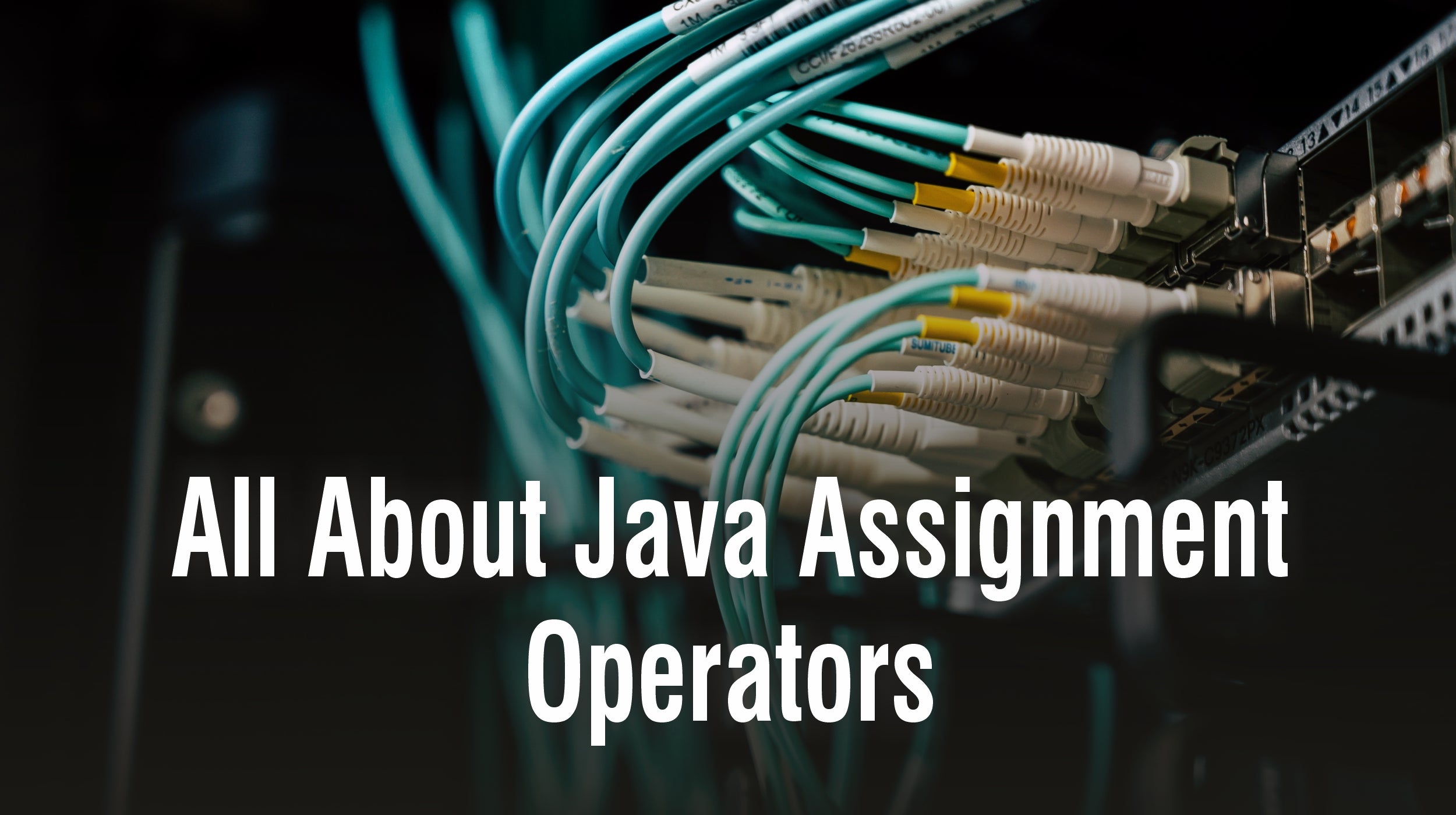
All About Java Assignment Operators
by Vibrant Publishers
Like every other programming language, Java language also provides a feature called Operators. There are a few types of Operators exist in Java. In this java tutorial, we will focus our learning on Assignment operators.
Assignment Operators exist for assigning a value to a variable in Java. The right side of the operator will be a value and the left side will be the variable that we created. So a general syntax would be as follows:
VARIABLE assignment operator VALUE ; |
They are also known as Binary Operators because it requires two operands for it to work. Since there are many data types in java, one important thing here to remember is that the data type of the variable must match with the value that we assign. These variables can be a java array variable, a java object or any variable of a primitive data type.
Now let’s look into multiple assignment operators that exist in Java language.
Simple Assignment Operator:
As the name suggests this operator assigns values to variables.Syntax:
variable = value ; |
Example:
string name = “This is awesome!”; |
A sample java code will be as given below:

Apart from simple assignment operations, java developers use this operator combined with other operators. This gives compound operators in the java code world. Let’s see those operators here:
+= Assignment Operator:
This is a combination of + and = java operators. So instead of writing,
number = number + 10; |
we can combine the above statement in a java code as:
number += 10; |
For Example:
The given java code blocks for java compiler will be the same and will produce the same output ie. 13.2
float java = 1.00; java +=12.2; float java = 1.00; java = java + 12.2; |
-= Assignment Operator:
Similar to the compound statement that we saw above, here also we combine ‘-’ and ‘=’ java operators. So here the value on the right side is subtracted from the initial value of the variable and it is assigned to the variable itself.Syntax:
numberA -= numberB ; |
Example:
numberA -= 10; this means numberA = numberA -10; |
*= Assignment Operator:
In this case, we combine ‘*’ and ‘=’ java operators. So here the value on the right side is multiplied with the initial value of the variable and it is assigned to the variable itself.Syntax:
numberA *= numberB ; |
Example:
numberA *= 10; this means numberA = numberA *10; |
/= Assignment Operator:
Just like the above cases, here we combine ‘/’ and ‘=’ java operators. In this operation, the initial value of the variable on the left side is divided with the value on the right side and the resulting quotient is assigned to the left side variable itself.Syntax:
numberA /= numberB ; |
Example:
numberA /= 10; this means numberA = numberA /10; |
Summary:
The following table provides a summary of the Assignment Operators in Java Language.

For a java developer, it’s important to understand the usage of Operators. If you want to learn java programming, then you must know these concepts well.
Share