Home
-
Blogs On Job Interview Questions
-
Do you need to prepare for C/C++ Interview Questions and Answers?
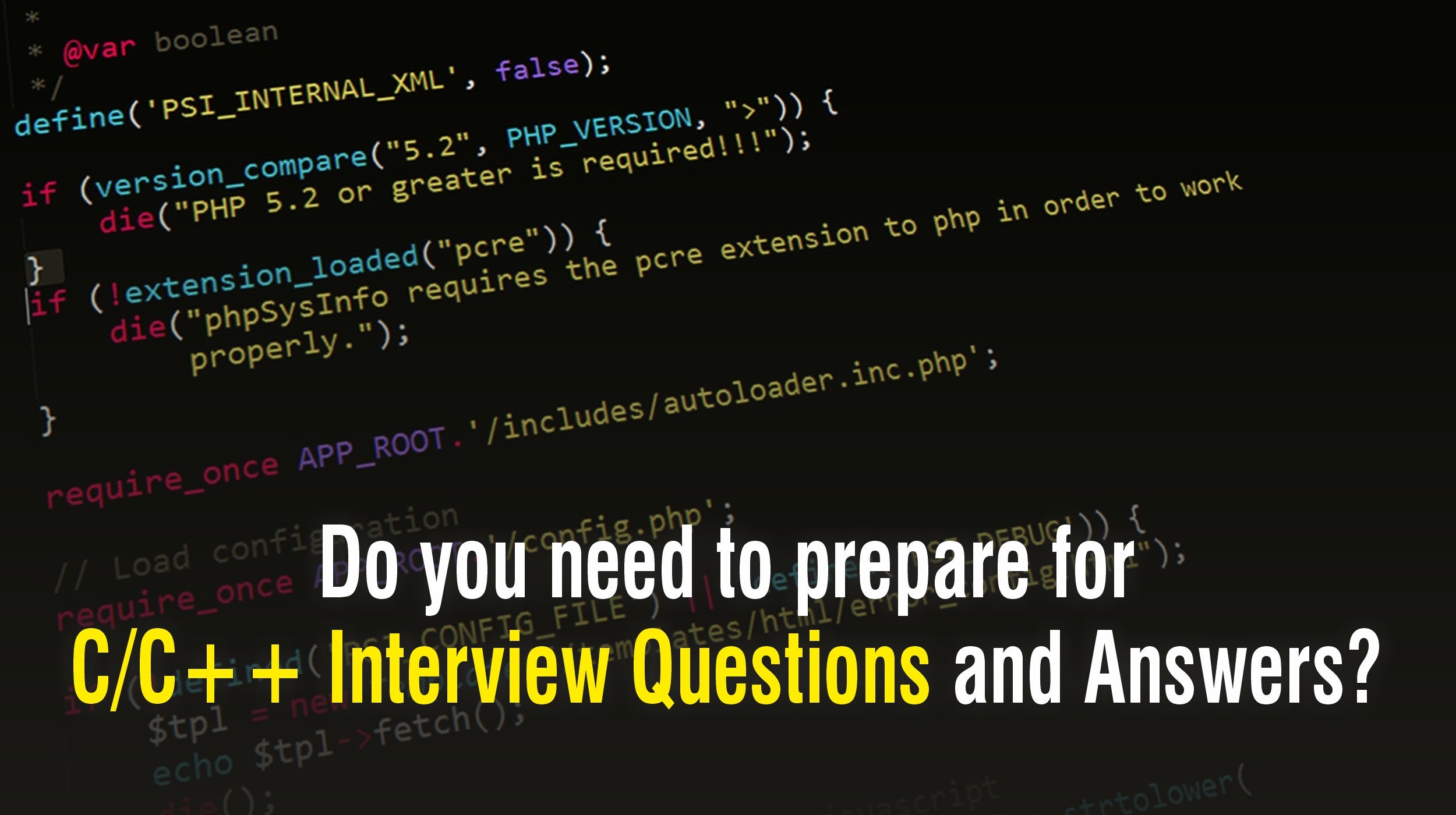
Do you need to prepare for C/C++ Interview Questions and Answers?
by Vibrant Publishers
With modern programming languages like Java, Python, and PHP being used for most applications, C/C++ programming languages sound outdated.
The above program will give a compilation error since inside a structure or class declaration the variables can’t be initialized. Only through structure variables can the members of a structure be initialized.
The program prints the first 15 numbers in the Fibonacci series.
But being outdated doesn’t mean it is not important!
C/C++ forms the basis of other languages and learning them is a prerequisite for understanding and efficiently coding in any other modern programming language. Remember, the interpreters and compilers of modern programming languages are written in C/C++.
C tackles all types of system software while C++ facilitates real-time simulation hence creating huge demand for them in areas such as game development or for quick access to codes to improve application efficiency as these codes work closely with the performance of the hardware.
Companies such as Gameloft, Accenture, Wipro are always on the lookout for skilled enthusiasts in C/C++ and provide for attractive job conditions.
No matter which programming language is used for developing applications, Interviewers always ask questions on C/C++ Programming as they want to hire the most knowledgeable and updated candidates who are well-versed with the latest changes and trends in programming languages. The questions may come from a wide range of topics like complex functions, data models, arrays, and general logic-based questions, and at times they can be very tricky too.
So how do you know what kind of questions to prepare for?
Below are some questions interviewers may ask during an interview.
1) What are Bit Fields?
Bit fields in C let the programmer specify the actual data size allowed for the particular member. Even if an unsigned int type is declared, the memory allocated is 4 bytes irrespective of whether or not the data will occupy that much space in memory. Bit fields let you specify the maximum size to be allocated in the memory which optimizes the program. The bit fields also can be used to force alignment of memory allocated. Bit fields cannot be assigned to pointers. It can be used to implement a boundary to the value assigned during the runtime.
2) What is a nested structure?
A structure declaration can contain a structure along with other data types, and this is called nesting structures. There are two ways this can be achieved. One is to put the structure declaration inside another structure declaration along with other data types. The second is to first declare the structure that is going to be embedded and then simply declare the already created structure inside the newly created structure along with other data types.
3) Explain the output of the following program.
void main () { struct student { int roll_No= 06123; char student_name = ‘Xyz’; char section = ‚A‛ ; }; } |
The above program will give a compilation error since inside a structure or class declaration the variables can’t be initialized. Only through structure variables can the members of a structure be initialized.
4) What is function overloading in C++ and how does it differ from function overriding?
When more than one function in a module has the same name but they differ in their signature, then it is called function overloading. The Signature of a function includes type of arguments passed to a function as well as number of arguments passed. In C++, return type of a function can’t be considered as a way to implement function overloading in a program, but in C it is possible. Function overriding is seen in inheritance, when two classes are defined and one is inherited from the other and both carry the same function name including same signature and return type. The logic implemented inside those functions may vary, though it is not mandatory.
5) Write a program to print the Fibonacci series using recursive function.
The program prints the first 15 numbers in the Fibonacci series.
#include <stdio.h> int fnFibonacci(int num) { if(num == 0) return 0; if(num == 1) return 1; return fnFibonacci(num-1) + fnFibonacci(num-2); } int main() { for (int num = 0; num < 15; num++) { printf(“%d\n”, fnFibonacci(num)); } return 0; } |
6) What are the advantages and disadvantages of using recursion?
An advantage of using recursion is the simplicity. The complex coding using iterative statements can be solved in a simple way using recursion.The disadvantage of using recursion is that every time it is required to save the return address, function parameters, etc., are in the stack for each function call.
7) Explain Multiple Indirection in C++.
Having a pointer to a pointer in C++ is possible. This is called Multiple Indirection. Normally a pointer contains the address of the variable that it points to. But in Multiple Indirection, one pointer contains the address of another pointer that contains the address of the variable. Even though, multiple indirection can be implemented upto any levels, a pointer to pointer is enough to handle most of the situations. When you have multiple levels of multiple indirections it becomes very difficult to track. A pointer to a pointer must be declared as <datatype> **<pointername>. When you declare a pointer to a pointer as int **pptr, it means that pptr is a pointer to an int pointer rather than pointer to an int.
8) Why do we need templates in C++?
Templates in C++ can be used for many important purposes. Since they are generic functions, they can be used to create type-safe stacks. Templates reduce the code. Since Templates can handle all data types, you can create any type of collection class using a Template. They are also used to implement smart pointers to hide the operator overriding techniques. Templates being classes, encapsulate the operator overriding details. You can also implements extra type-checking with templates. Even though these functionalities can be implemented in many other ways also, Templates are much preferred as it is a write-once-work-with-all-datatypes method. They are easier to understand and help encapsulation. Since the compiler can determine the data type during compile time, Templates are more typesafe.
9) What is the role of linker in C?
After the compiler produces files containing machine understandable object code, the linker takes those object files along with predefined library functions used in the program and produces the final executable file. Now the executable file can be executed using loader.
10) What is a register variable and when it can be used?
A register variable is a special type of variable where memory is allocated in the CPU registers. It can be declared by using the keyword ‘register’.The advantage of declaring a register for a frequently employed variable is to speed up the execution of the program. The number of register variables in a program is limited. When compiler cannot allocate a register variable due to limited availability of CPU registers, then the variable is allocated as auto variable.
11) What is the difference between the way variables are defined in C and C++?
It is compulsory that local variables have to be defined at the start of a block or a function or a program in C, where as in C++, the local variables can be defined at any point inside a block or a function giving the flexibility to the programmer to declare and use the variable at any point.
12) Why are variables declared?
Declaring a variable serves two main purposes:-
During declaration of a variable the type of data the variable is going to hold is known to the compiler
-
A name is associated to the variable so that variable can be easily accessed using this name
13) What is operator precedence?
Operator precedence defines the order operators are used in an expression, and it follows typical algebraic precedence. For example, the expression ‘a+b*c’ is evaluated as ‘a+(b*c)’ according to the operator precedence rule (since multiplication takes precedence over addition).
14) What is the difference between exit() and abort() functions in C?
The exit() function systematically exits the program after closing all the open files, flushing the buffer clear, removing any temporary files and also returns an exit status whether or not the exit was successful. When you call an exit() function, it performs any instructions specified in the at exit() function. The exit() is an elegant way of exiting the program. The abort() abruptly ends the program. It does not bother to close the open files or delete the temporary files created by the program. It will not perform the instructions given in the at exit() function. In fact, it will just abort the program. The only way to handle an abort() is to include a SIGABRT signal handler.
15) What is the use of heap in C program?
Heap refers to a memory area where variables created dynamically are stored. Generally, a pointer is declared to track the dynamic memory location of a variable. Apart from these, global and static variables are also created in the heap area of main memory.
16) What is a constructor and what is its primary function?
A constructor is a special type of method that can be defined inside a class and this is the first method to be called while an object is created for a class. It is of two types, default constructor and parameterized constructor.The primary function of a constructor is to initialize the data members when an object of a class is instantiated. If arguments are passed to the constructor, then it is called a parameterized constructor. If the constructor doesn’t take any parameters, then it is called a default constructor. Also, another type of constructor is the dummy constructor, which is not defined inside the class. It is the responsibility of the complier to call a dummy constructor.
17) What is Multiple inheritance and how does it differ from Hierarchical inheritance?
Multiple inheritance is a form of inheritance where a child class is derived from more than one base class; for example a child class can have more than one parent class. But in case of Hierarchical inheritance, more than one child class is derived from a single parent class. Therefore Multiple and Hierarchical are the two opposite forms of inheritance.
18) When do static members can come in handy while coding in C++?
When the programmer wants to keep track of a variable for all the objects of a class (like calculating how many objects are still alive or how many total objects have been created so far), it is accomplished by implementing static members for a class.
19) What is dynamic object in C++?
Like dynamic memory allocation for data variables at run time, a class can also be initialized or instantiated at run time by using the ‘new’ operator and those objects are called ‘dynamic objects’. Like dynamic memory allocation for normal data variables, dynamic objects also allocate memory from heap.
20) What is constructor overloading in C++?
A class can have a member function whose name matches the name of the class itself, and that member function is called constructor. A class can have more than one such function that differs only in terms of their signature, i.e. types of arguments accepted by those functions or number of arguments being accepted by them or both.Always remember the more questions you solve in practice, the better will be your chances for clearing the interview. These interview questions are sure to fill you with confidence to help you land your dream job!
All the best!
Share